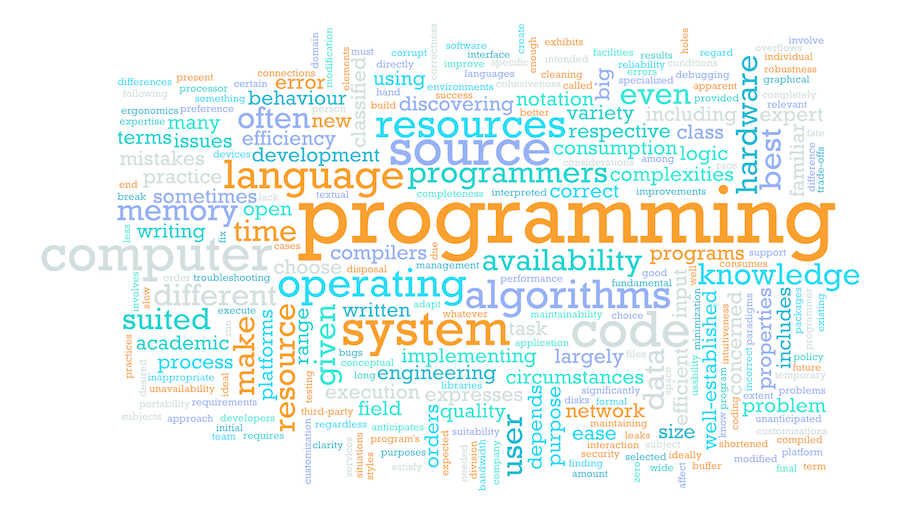
In real life, learning another languages will help you better know and feel the culture, the lifestyle, and so. As a result, we get to love the language, the people and the country, right? Similarly, learning the most basic programming terms will allow you to understand and utilize your knowledge into real life job. After all, no developers or programmers that don’t study these basic programming terms.
For starters, don’t worry because this article is dedicated to you. AHT Tech will slowly but surely guides you through key terms in the alphabetical order.
Prepare a pen and a notebook to jot down any points that you haven’t known yet. And now, let’s get started!
Basic programming terms that start with A, B, C, D
Algorithm
Algorithm is a set of rules or requirements used to solve a particular problem. The complexity can go from adding 2 numbers, to converting between different formats.
Algorithm in programming:
my $word = "hope"; my $n = 0; my @words = split(//, $word); foreach (@words) { $n++; } print "Letters: $n";
API – Basic programming terms
API stands for Application Programming Interface. It is a set of routines and protocols to build apps. API programmers can easily access other companies’ program or service. For this reason, big companies such as Facebook or Twitter all use APIs to let their employees get better access to their own programs.
Argument
Argument or arg is one of the basic programming terms that refers to a command line. The example below is the argument for the edit command:
edit myfile.txt
Let’s take another example. If the routine is to square up the number, then:
SQR(4) = 16
ASCII
ASCII is short for American Standard Code for Information Interexchange. Out of topic, but it’s pronounced “as-key”. It is a standard format of binary or 8-bit code. For example, “h” in ASCII has the decimal value 104, and 0110100 in binary. There are only 256 available slots. To learn more, go to ASCII table right here!
Array
Array is quite similar to a range. It can be listed as groups of similar data or values. For anyone using Excel, Array is one of the very familiar basic programming terms. Take the number of students for example, if each student is referred to a number (value) then the whole class would be an array. Here is a JavaScript array example:
function myFunc(){
var numbers = ["one", "two", "three", "four", "five"];
numbers.toString();
document.getElementById("example").innerHTML = numbers[0] + " and " + numbers[1] + " equals 3"; }
Backend – basic programming terms
As the name suggests, backend tasks refer to what is performed in the background or indirectly. A backend developer will design the program and process the data underneath what everyone can see.
Boolean
This basic programming terms refer to a data type that displays only 2 possibilities: true or false. If you look further, Boolean expressions use AND, OR, XOR, NOT and NOR operators with conditional statements. Let’s have a look at the following example of if statement in Boolean:
use strict; my ($name, $password); print "\nName: "; chomp($name = <STDIN>); print "\nPassword: "; chomp($password = <STDIN>); if (($name eq "bob") && ($password eq "example")) { print "Success\n"; } else { print "Fail\n"; die; }
Bug
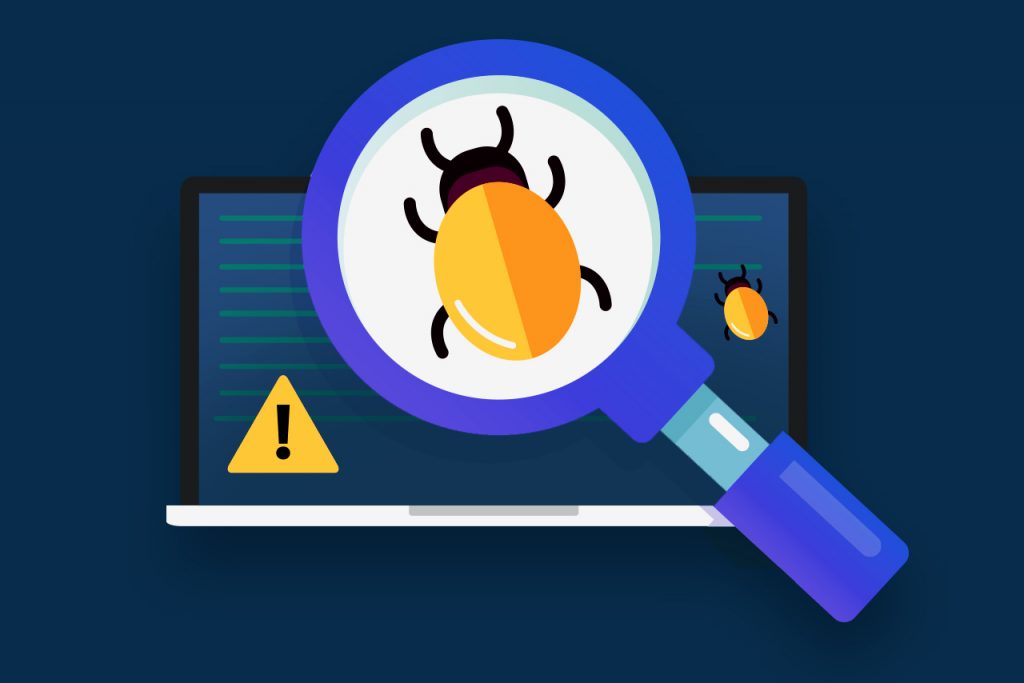
Bug is a basic programming terms used to describe unexpected hardware or software issues. Most bugs lead to minor problems, but some can cause irreparable damage. People might mistake an error with a bug. To compare, an error is the message to show the users what is wrong. On the other hand, a bug is the problem that causes the error.
Char
Char is short for Character. Familiar now? Char is mainly used in programming languages (C, C#, C++, Java, etc.) It is a data type that has one character as 1 letter, number or so only.
Class
This basic programming terms refer to a set of linked elements with common features. Class is super important in creating a powerful and flexible object-oriented programming. A class could be number, shape, people, … anything has common characteristics.
Code – basic programming terms
Code is a term used to describe instructions or rules via a particular programming language namely Java, Python or C. We categorize codes by the used-language. For example: PHP Code, HTML Code, CSS Code or Java Code. Here is an example of C# Code which prints AHT Tech :
public class AHT Tech { public static void Main() { System.Console.WriteLine("AHT Tech"); } }
Command-line Interface
It refers to a user interface based on the text. Some other similar basic programming terms are console user interface or character user interface. In history (60s to 80s), command-line interface was the common way to interact with computers.
Compilation
Compilation is a process when a computer converts a high-level programming language (which AHT will describe later) into a machine language. The computer that executes compilation is called a compiler.
Conditionals
To help the code decide “True or False” statements, the programming language must have conditionals, conditional statements as well as conditional expressions. Each will perform different acts and serve multiple purposes. Some example of conditional statements are:
IF a value is less than 10, THEN display the words "Value is less than 10" on the screen. if ($myval < 10) { print "Value is less than 10"; }
Constant – basic programming terms
… or Const is a common term. A constant is a never-changed value or number in a specified period of time. Thus, it cannot be modified or altered. How to define a constant? Check out this example:
define ('EXAMPLE', 'Breakfast\n'); echo 'I’m hungry? ', EXAMPLE;
Data Type
Data Type is basic programming terms referring to the classification of data. A computer doesn’t know the differences between numbers and letters, so it will need a data type to categorize variables. Some usual data types are:
- Boolean (we have mentioned above): True, False,…
- Character: a, b, x, y, …
- Date
- Double
- Floating-point number: 9.876
- Integer
- Short
- Long
- String: xyz
- Void: no data
Dart
Dart is an open-source web-based programming language developed by Google. We did have a very dedicated article on Dart that you cannot miss out on!
Dart: what is it and why Flutter uses Dart?
Debugging
Debugging is a basic programming terms that refers to an analytical process to remove errors. If you are using Node.js, don’t forget to check this article:
Debugging node js apps: Ultimate 9 tips and tricks for Node.js application
Debugger
It could be a developer or a tool that is able to locate errors and issues within the computer’s program.
Declaration
… is a statement that describes a variable or a function. Its purpose is to identify a word and the meaning to continue the process. Depending on which programming language is used, a declaration can required or optional:
use strict; my $help;
Basic programming terms that start with E, F, I, K, L, M
Exception
Exception is a term that refers to unexpected or special acts. It can be errors, conditions and so. Commonly, the exception must be expected beforehand, and will propose some solutions. The basic programming terms for that preparation is called exception handling.
Expression
Expression is a combination of numbers, symbols and letters to represent a variable ( or a value). For each expression, there is one or lots of operands (we will cover this programming term later) and operators (again, we will go through it afterwards).
Framework – basic programming terms
We all heard of frameworks many times before, but what exactly are they? A framework has tons of APIs, software libraries, compilers and so many more. It provides the perfect environment for a particular software development project.
AHT has some useful articles for these frameworks that you should not ignore!
- Java Framework
- PHP Framework
- Express Framework
- Node.js Framework
- And many more at our blog site!
Front-end
Front-end is an opposite programming term to “backend”. It is the user interface of a device. Thus, Front-end developers are those who develop and design UI.
Hardcode
Hardcode or Hard code refers to hardly changeable codes. It was originally built for fixed codes in software or hardware programming. Some developers consider Hardcode the most difficult language to learn.
High-level language (HLL) – basic programming terms
… refers to a programming language that allows developers to write programs that aren’t limited by the computer. As a result, it is much easier to understand, because it gets more “human” and less “machine”. Some common HLL are C++, C, Java, PHP, Python, Ruby, BASIC,…
IDE
IDE is short for Integrated Development Environment. IDEs are visual tools that boost a program’s efficiency. Here are the top 20 IDEs that most developers are using these days!
Best IDE for web development : Top 20 to create websites of any complexity
Iteration
This is a basic programming terms that refers to a single pass through operations that deal with multiple codes. Loop is a common form of iteration. To clarify, an iteration is the process of repeating codes for a particular purpose.
Keywords
Yes, Keywords is one of the most common basic programming terms that you must have known. But in programming, keywords refer to a special case that can perform specific tasks. Moreover, each programming language all has different sets of keywords that cannot be used as variables.
Loop
Loop refers to a program that repeats the same information all the time until being told to stop. Loops are one of the most basic yet powerful concepts in programming.
Low-level language (LLL)
Low-level language is the opposite of high-level language. It resembles machine language more, so it is quite difficult for even experienced developers, too.
Machine language – basic programming terms
… or Machine code, it’s the lowest-level programming language (yes, lower than low-level language). It only consists of binary digits (0&1) and bits. As a result, only computers can read machine language. How can we learn it? Programmers would write codes in high-level language then translate it into machine language.
For example, this is “Hello World”
01001000 01100101 01101100 01101100 01101111 00100000 01010111 01101111 01110010 01101100 01100100
Mark-up language
Mark-up language is one of the most simple basic programming terms. It consists of common keywords, tags and names to help users easily know the data content. Some popular examples are XML, HTML, BBC and SGML.
Terms that start with N, O, P, R, S, T, V
Null
Null is a term that defines a value lack. It can be a programming code, missing value,… Take this as an example:
my $value1 = “”;
my $value2 = “1”;
$value1 var is null, and $value2 has a value of 1.
Object
Object is the combination or constants, variables or other data that are closely related. Furthermore, it can include shapes or numbers (age, height,…).
Object-Oriented Programming (OOP) – basic programming terms
This programming term refers to a model that goes around the objects and data. It can be structured as reusable elements with shared properties. Its purpose is to extend functionalities and refactor and maintain the codes.
Operand
… is a programming term used to describe a manipulated object (if possible).
Operator
… is a programming term used to describe an object that can manipulate a value.
An example of operand and operator: if 1, 2 and 3 is an operand, then in the command “1+2=3”, the plus and equal sign is an operator.
Package
Package is a wider range for classes. Its purpose is to organize classes with similar functionality and categories.
Pointer
In programming, a pointer refers to an address in memory of other variables. With a pointer, the performance of the program can be highly improved as it is cheaper in time and space to copy.
Program
Program is one of the most basic programming terms, right? A program is normally processed by the CPU before being executed. Web browsers also use programs to let us browse the Internet.
Runtime
This term describes the time that a program is running on a computer. Simple as that!
Server-side
Server-side is contrast to client-side. When some processing methods such as calculations, functions are executed on a server, it is called server-side. Some common examples are shopping carts and search engines.
Source data
… or data source is the location where the data is used in the program. It can be the database, dataset, spreadsheet or hard coded data. The program will get the data from its source then displays it in the code.
SQLite – basic programming terms
SQLite is a very Lightweight relational database management system (RDBMS) for SQL. Its purpose is to speed up the performance and add database functionality. For more information, check out our use article right below:
SQLite: What is it and how to use it in Flutter?
Statement
Statement is a programming term that refers to a single code line that instructs or expresses an action. Each statement may have internal components of its own such as expressions, operators and functions.
Syntax
Syntax is a set of rules for ways of conveying statements. Even if some programming languages have many features, functions in common, they all differ in syntax. What’s more, a faulty syntax can lead to a chain of errors.
Token
Token is the smallest unit possible in a program. In today’s date 10/14/20, the token would be 10, 14, and 20. By gathering tokens, the programmer can set up any portions they need.
Variable
… is a named unit of data. We all learn about variables in Math, so you must have known about it. In programming, some variables are mutable or changeable. And some are immutable, meaning they cannot be changed or deleted.
Want to know more basic programming terms? Contact us NOW!
AHT Tech is proud to be one of the leading IT Outsourcing agencies in Vietnam. With over 14 years in the industries, satisfying more than 10,000 clients globally, we are confident that your issues will be solved in no time! Our services and solutions are fast, low cost and always bring a smile to all clients’ face!
Wrapping Up
Thank you so much for joining us on the topic of top 50 basic programming terms and definitions today. AHT Tech hopes that you find it helpful for your journey.
And as always, we truly wish the best of luck and success will come to you! WE’D LOVE TO HEAR FROM YOU AT: Contact Us Form